yade.bf module¶
Overview¶
This module contains breakage functions (bf) that can be used for particle breakage by replacement approach. Functions can be used for both spheres and clumps of spheres. However, this module is particularly useful for clumps because it deals with multiple clump-specific issues:
Clump members do not interact. Hence, modification of the Love-Webber stress tensor is proposed to mimic interactions between clump members when the stress state is computed.
If clumped spheres overlap, their total mass and volume are bigger than the mass and volume of the clump. Thus, clump should not split by simply releasing clump members. The mass of new sub-particles is adjusted to balance the mass of a nonoverlapping volume of the broken clump member.
New sub-particles can be generated beyond the outline of the broken clump member to avoid excessive overlapping. Particles are generated taking into account the positions of neighbor particles and additional constraints (e.g. predicate can be prescribed to make sure that new particles are generated inside the container).
Clump breakage algorithm¶
The typical workflow consists of the following steps (full description in [Brzezinski2022]):
Stress computation of each clump member. The stress is computed using the Love-Weber (LV) definition of the stress tensor. Then, a proposed correction of the stress tensor is applied.
Based on the adopted strength criterion, the level of effort for each clump member is computed. Clump breaks if the level of effort for any member is greater than one. Only the most strained member can be split in one iteration.
The most strained member of the clump is first released from the clump and erased from simulation. New mass and moment of inertia are computed for the new clump. The difference between the “old” and the “new” mass must be replaced by new bodies in the simulation.
New, smaller spheres are added to the simulation balancing the mass difference. The spheres are placed in the void space, hence do not overlap with other bodies that are already present in the simulation (splitting_clump).
Finally, the soundness of the remaining part of the original clump needs to be verified. If the clump members do not contact each other anymore, the clump needs to be replaced with smaller clumps/spheres (handling_new_clumps).
Optionally, overlapping between new sub-particles of sub-particles and existing bodies can be allowed (packing_parameters).
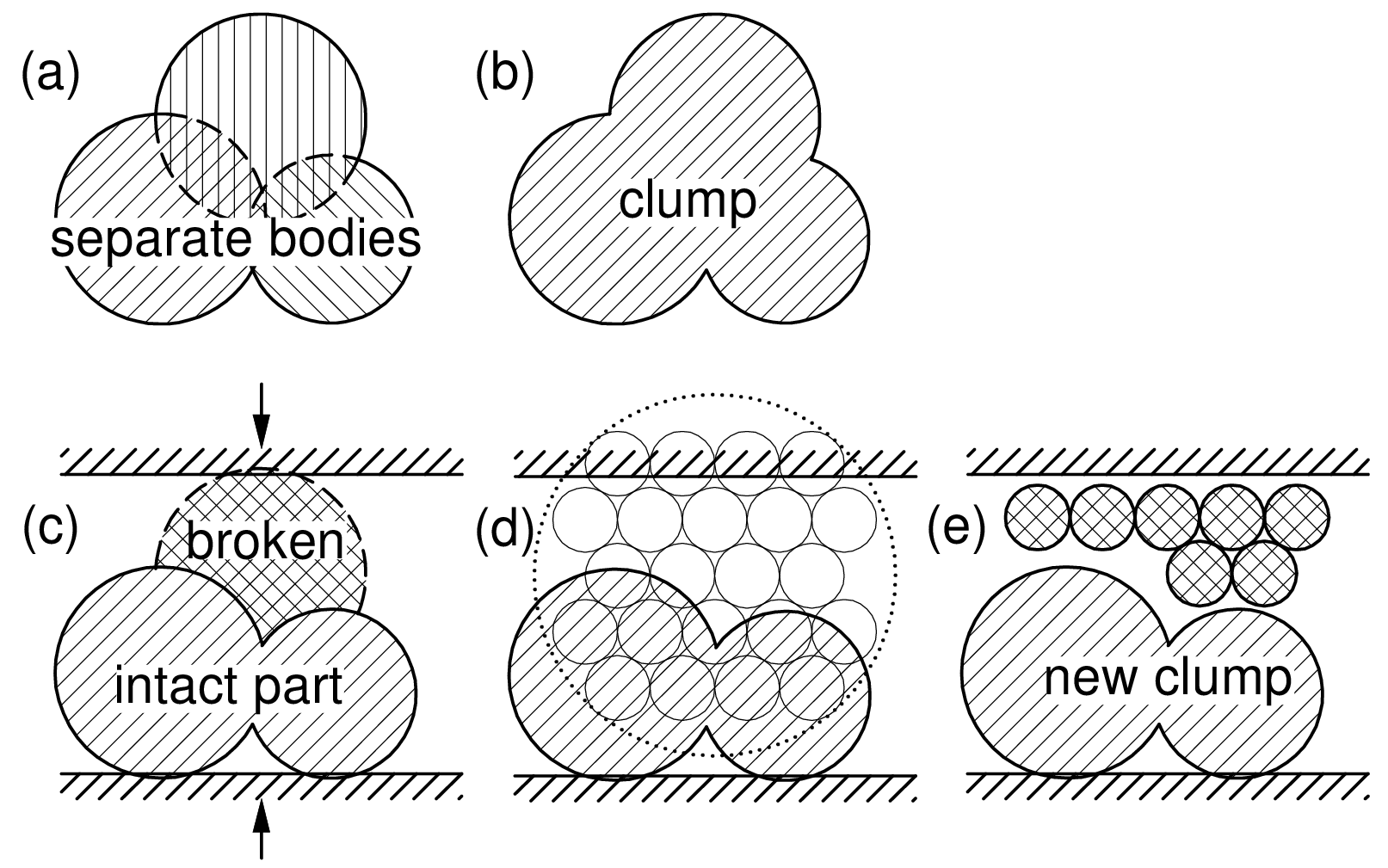
Stages of creating a clump in Yade software and splitting due to the proposed algorithm: (a) overlapping bodies, (b) clumped body (reduced mass and moments of inertia), (c) selection of clump member for splitting, (d) searching for potential positions of sub-particles, (e) replacing clump member with sub-particles, updating clump mass and moments of inertia.¶
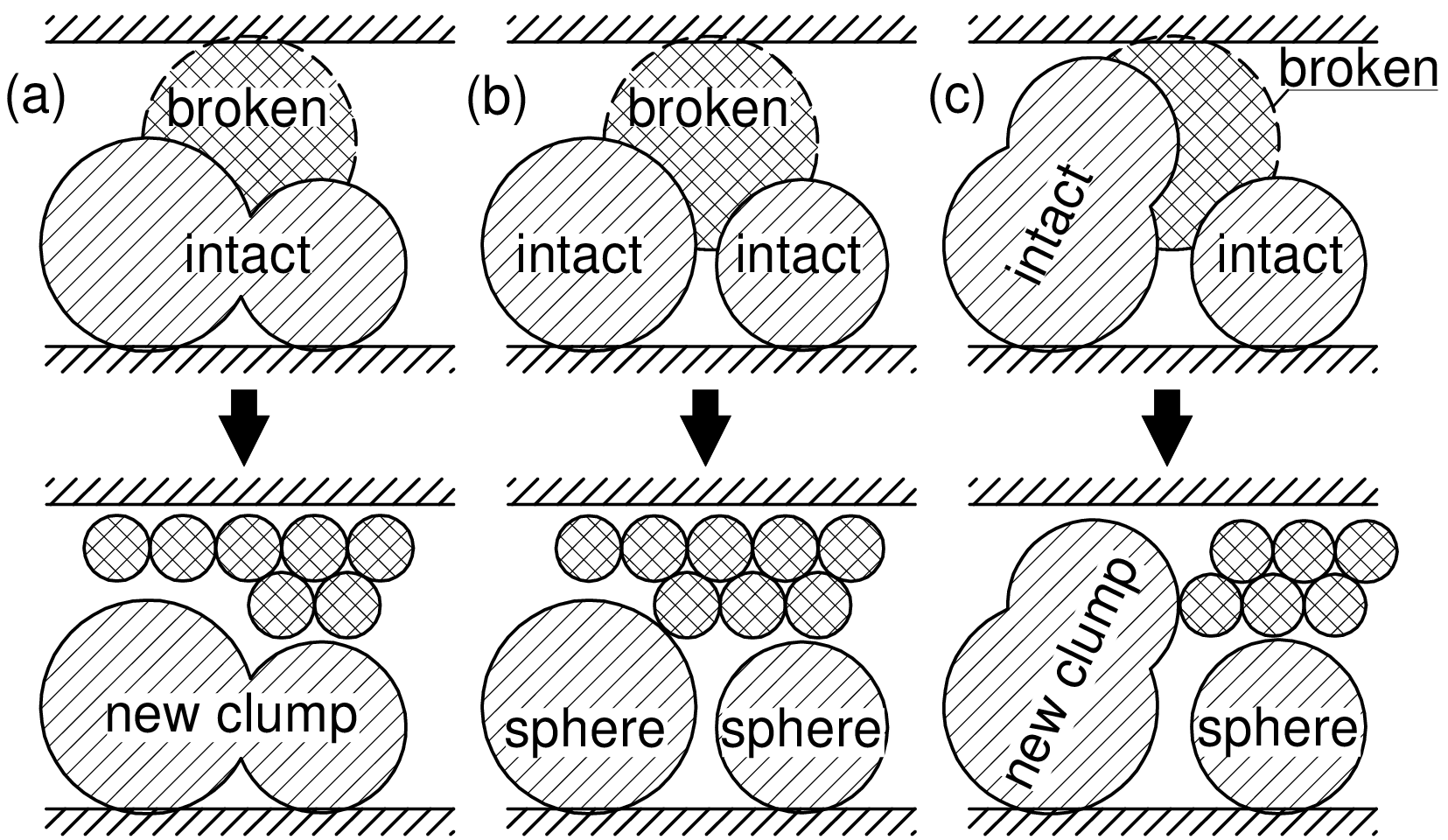
Different scenarios of clump splitting: (a) clump remains in the simulation - only updated, (b) clump is split into spheres, (c) clump is split into a sphere and a new clump.¶
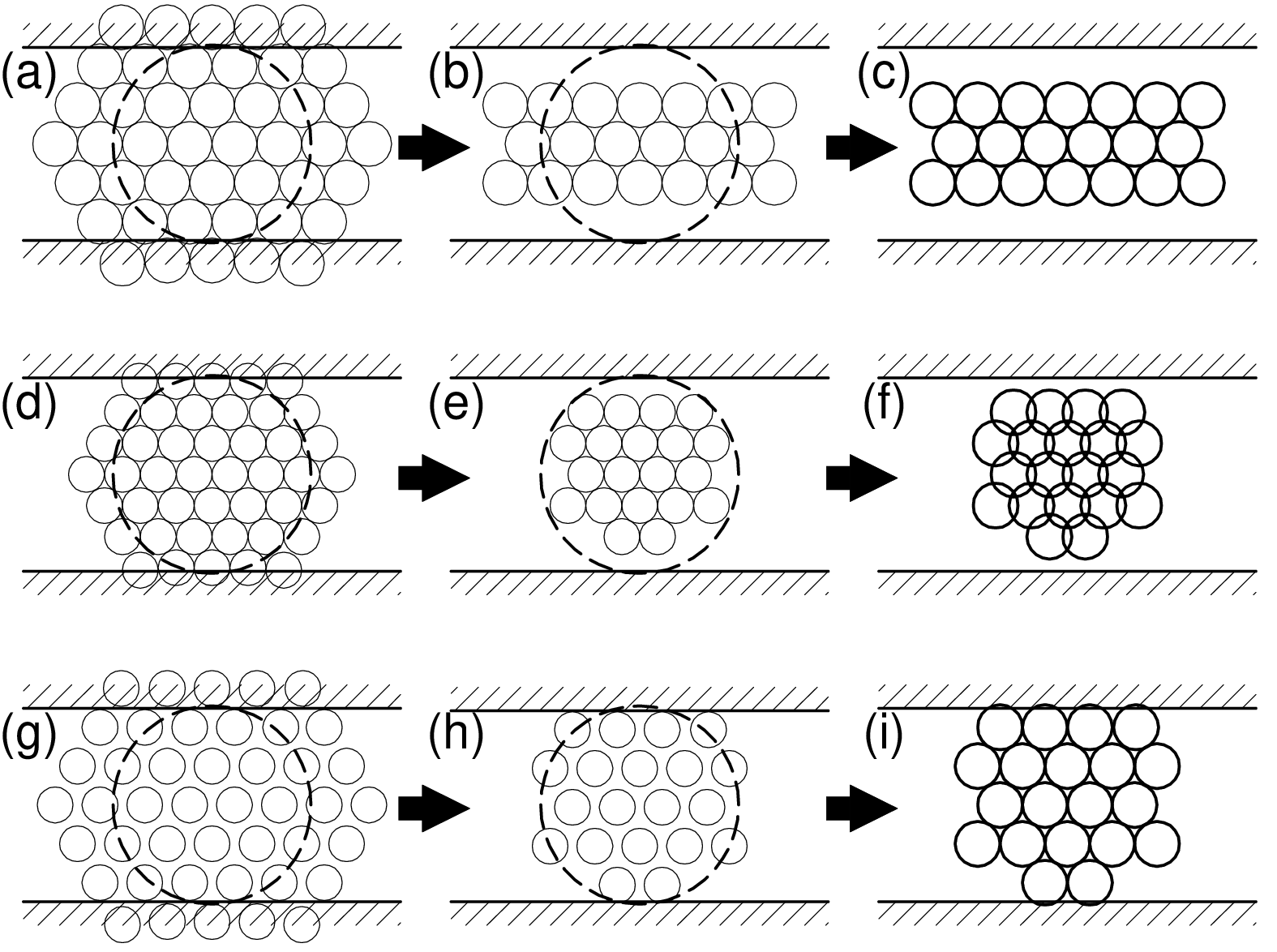
Replacing sphere with sub-particles: (a-c) non-overlapping, (d-f) overlapping sub-particles and potentially overlapping with neighbor bodies, (g-i) non-overlapping sub-particles but potentially overlapping with neighbor bodies.¶
- Functions required for clump breakage algorithm described in:
Brzeziński, K., & Gladky, A. (2022), Clump breakage algorithm for DEM simulation of crushable aggregates. [Brzezinski2022]
- Strength Criterion adopted from;
Gladkyy, A., & Kuna, M. (2017). DEM simulation of polyhedral particle cracking using a combined Mohr–Coulomb–Weibull failure criterion. Granular Matter, 19(3), 41. [Gladky2017]
- yade.bf.checkFailure(b, tension_strength, compressive_strength, wei_V0=0.01, wei_P=0.63, wei_m=3, weibull=True, stress_correction=True)[source]¶
Strength criterion adopted from [Gladkyy and Kuna 2017]. Returns particles ‘effort’ (equivalent stress / strength) if the strength is exceeded, and zero othervise.
- yade.bf.evalClump(clump_id, radius_ratio, tension_strength, compressive_strength, relative_gap=0, wei_V0=0.001, wei_P=0.63, wei_m=3, weibull=True, stress_correction=True, initial_packing_scale=1.5, max_scale=3, search_for_neighbours=True, outer_predicate=None, discretization=20, grow_radius=1.0, max_grow_radius=2.0)[source]¶
Iterates over clump members with “checkFailure” function. Replaces the broken clump member with subparticles. Split new clump if necessary. If clump is not broken returns False, if broken True.
- yade.bf.replaceSphere(sphere_id, subparticles_mass=None, radius_ratio=2, relative_gap=0, neighbours_ids=[], initial_packing_scale=1.5, max_scale=3, scale_multiplier=None, search_for_neighbours=True, outer_predicate=None, grow_radius=1.0, max_grow_radius=2.0)[source]¶
This function is intended to replace sphere with subparticles. It is dedicated for spheres replaced from clumps (but not only). Thus, two features are utilized: - subparticles_mass (mass of the subparticles after replacement), since in a clump only a fraction of original spheres mass is taken into account - neighbours_ids - list of ids of the neighbour bodies (e.g. other clump members, other bodies that sphere is contacting with) that we do not want to penetrate with new spheres (maybe it could be later use to avoid penetration of other bodies). However, passing neighbours_ids is not always necessary. By default (if search_for_neighbours==True), existing spheres are detected authomatically and added to neighbours_ids. Also, outer_predicate can beused to avoid penetrating other bodies with subparticles. Spheres will be initially populated in a hexagonal packing (predicate with dimension of sphere diameter multiplied by initial_packing_scale ). Initial packing scale is greater than one to make sure that sufficient number of spheres will be produced to obtain reguired mass (taking into account porosity). scale_multiplier - if a sufficient number of particles cannot be produced with initial packing scale, it is multiplied by scale multiplier. The procedure is repeated until initial_packing scale is reached. If scale_multiplier is None it will be changed to max_scale/initial_packing_scale, so the maximum range will be achieved in second iteration. max_scale - limits the initial_packing_scale which can be increased by the algorithm. If initial_packing_scale >max_scale, sphere will not be replaced (broken). outer_predicate - it is an additional constraint for subparticles generation. Can be used when non spherical bodies are in vicinity of the broken particle, particles are in box etc. search_for_neighbours - if True searches for additional neighbours (spheres whithin a range of initial_packing_scale *sphere_radius)
Particles can be generated with smaller radius and than sligtly growed (by “grow_radius”). It allows for adding extra potential energy in the simulation, and increase the chances for successful packing. relative_gap - is the gap between packed subparticles (relalive to the radius of subparticle), note that if grow_radius > 1, during subparticles arangemenr their radius is temporarily decreased by 1/grow radius. It can be used to create special cases for overlapping (described in the paper).